Fundamentals Of AutoIt, Programming Language
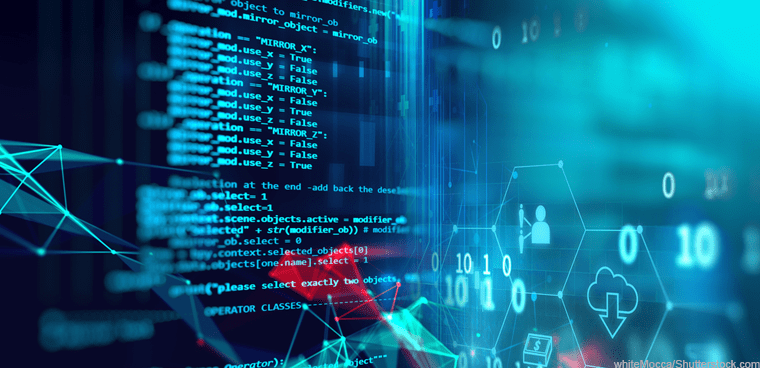
AutoIt is one of the Interpreted Programming Languages
What are Interpreted Programming Languages
An interpreted language is a programming language for which most of its implementations execute instructions directly, without previously compiling a program into machine-language instructions. The interpreter executes the program directly, translating each statement into a sequence of one or more subroutines already compiled into machine code. (Wikipedia)
Variables
What Are Variables
In computer programming, a variable or scalar is a storage location (identified by a memory address) paired with an associated symbolic name, which contains some known or unknown quantity of information referred to as a value or In easy terms, a variable is a container for different types of data (like integer, float, String etc…). The variable name is the usual way to reference the stored value, besides referring to the variable itself, depending on the context. This separation of name and content allows the name to be used independently of the exact information it represents. The identifier in computer source code can be bound to a value during runtime, and the value of the variable may thus change during program execution.
Variables in AutoIt Programming Language
Declarations
A variable is a placeholder in memory where we can store some kind of data. In AutoIt the data is a string, number or reference to an array. AutoIt has three keywords to declare a variable. Namely Global, Local and Dim. When we declare a variable we set aside some memory for the variable. All variable names in AutoIt starts with a $ sign. So they are easy spotted in the code.
1 | Global $gVar |
Comments
What Are Comments
In computer programming, a comment is a programmer-readable explanation or annotation in the source code of a computer program. They are added with the purpose of making the source code easier for humans to understand and are generally ignored by compilers and interpreters. (Wikipedia)
Comments in AutoIt Programming Language
Two types of comments exist in every language. Comments are simply lines/blocks of code that are meant as guidelines or notes to help understand what the code is doing.
Inline/Single-line comments may be added using a proceeding semicolon.
1 | ; This is a single-line comment |
Whereas multi-line comments are a little less intuitive but just as effective…
1 | #comments-start |
There’s also a shorthand method to this.
1 | #cs |
Data Types
What Are Data Types
In computer science and computer programming, a data type or simply type is an attribute of data that tells the compiler or interpreter how the programmer intends to use the data. Most programming languages support basic data types of integer numbers (of varying sizes), floating-point numbers (which approximate real numbers), characters, and Booleans. A data type constrains the values that an expression, such as a variable or a function, might take. This data type defines the operations that can be done on the data, the meaning of the data, and the way values of that type can be stored. A data type provides a set of values from which an expression (i.e. variable, function, etc.) May take its values.
Data Types in AutoIt Programming Language
In AutoIt, there is only one data type called a Variant. A variant can contain numeric or string data and it decides how to use the data depending on the situation it is being used in. For example, if you try to multiply two variants they will be treated as numbers, if you try to concatenate (join) two variants they will be treated as strings.
Some examples:
- 10 * 20 equals the number 200 (* is used to multiply two numbers)
- 10 * ”20” equals the number 200
- ”10” * ”20” equals the number 200
- 10 & 20 equals the string ”1020” (& is used to join strings)
If a string is used as a number, an implicit call to Number() function is done. So if it doesn’t contain a valid number, it will be assumed to equal 0.
For example,
10 * ”fgh” equals the number 0.
If a string is used as a boolean and it is an empty string ”” , it will be assumed to equal False (see below).
For example,
Not ”” equals the Boolean True.
Numbers. Numbers can be standard decimal numbers like 2, 4.566, and -7.
Scientific notation is also supported; therefore, you could write 1.5e3 instead of 1500.
Integers
Integers (whole numbers) can also be represented in hexadecimal notation by preceding the integer with 0x as in 0x409 or 0x4fff.
Strings.
Strings are enclosed in double quotes like ”this”. If you want a string to actually contain a double-quote use it twice like:
”here is a ”double-quote” – ok?” You can also use single quotes like ’this’ and ’here is a ’ ’single-quote’ ’ – ok?’
You can mix quote types to make for easier working and to avoid having to double-up your quotes to get what you want. For example, if you want to use a lot of double-quotes in your strings then you should use single quotes for declaring them:
’This ”sentence” contains ”lots” of ”double-quotes” does it not?’
is much simpler than:
”This ”sentence” contains ”lots” of ”double-quotes” does it not?’
When evaluated, strings can have Env variables or Var variables substitution according to Opt() function definition.
All AutoIt strings use UTF-16 (in fact and more precisely UCS-2) encoding.
Booleans
- Booleans are logical values. Only two Boolean values exist: True and False.
- They can be used in variable assignments, together with the Boolean operators And, Or and Not.
- Examples:
- $bBoolean1 = True
- $bBoolean2 = False
- $bBoolean3 = $bBoolean1 And $bBoolean2
- This will result in $bBoolean3 being False
- $bBoolean1 = False
- $bBoolean2 = Not $bBoolean1
- This will result in $bBoolean2 being True
- If Boolean values are used together with numbers, the following rules apply:
- A value 0 will be equal to Boolean False
- Any other number value will be equal to Boolean True
- Example:
- $iNumber1 = 0
- $bBoolean1 = True
- $bBoolean2 = $iNumber1 And $bBoolean1
- This will result in $bBoolean2 being False
- If you use arithmetics together with Boolean values (which is not advisable!), the following rules apply:
- A Boolean True will be converted into the numeric value 1
- A Boolean False will be converted into the numeric value 0
- Example:
- $bBoolean1 = True
- $iNumber1 = 100
- $iNumber2 = $bBoolean1 + $iNumber1
- This will result in $iNumber2 to be the numeric value 101
- If you use strings together with Boolean values, they will be converted as follows:
- A Boolean True will be the string value ”True?”
- A Boolean False will be the string value ”False”
- Example:
- $bBoolean1 = True
- $sString1 = ”Test is: ”
- $sString2 = $sString1 & $bBoolean1
- This will result in $sString2 being the string value ”Test is: True”
- The other way around however is different. When you use string comparisons with Boolean values, the following rules apply:
- Only an empty string (””) will be a Boolean False
- Any other string values (including a string equal ”0”) will be a Boolean True
Binary
Binary type can store any byte value. They are converted into hexadecimal representation when stored in a string variable.
Example:
$dBin = Binary(”abc”)
$sString = String($dBin) ; ”0x616263”
Pointer
Pointer types store a memory address which is 32bits or 64bits depending on if the 32bit or 64-bit of AutoIt is used. They are converted to hexadecimal representation when stored in a string variable. Window handles (HWnd) as returned from WinGetHandle() are a pointer type.
Variable created with DllStructCreate() can be used as pointer if a ’struct*’ type in the DllCall() is used.
A pointer defined by DllStructGetPtr() can be passed to such parameter with a ’struct*’ type.
Data types and Ranges
The following table shows the internal variant datatypes and their ranges.
Data Sub-type | Range and Notes |
---|---|
Int32 | A 32bit signed integer number. |
Int64 | A 64bit signed integer number |
Double | A double-precision floating point number. |
String | Can contain strings of up to 2147483647 characters. |
Binary | Binary data, can contain up to 2147483647 bytes. |
Pointer | A memory address pointer. 32bit or 64bit depending on the version of AutoIt used. |
Initializations
What Are Initializations
In computer programming, initialization is the assignment of an initial value for a data object or variable. The manner in which initialization is performed depends on the programming language, as well as the type, storage class, etc., of an object to be initialized. (Wikipedia)
Initializations in AutoIt Programming Language
Assigning a value to a variable is the only way to get things going. Fortunately, AutoIt uses loose typing…
1 | Local $x = "Hi there!" ; $x is a string |
Loose typing simply means a variable is malleable and its format can be changed to your liking. This also means that you have to watch your scope and what’s going in and out of that variable.
Arrays are handled a bit differently. They may contain several types and those types are loosely typed but the array must have a predetermined size.
1 | Local $y[3]$y[0] = 1 ; first address is an integer |
Equivalently
1 | Local $z[3] = [1,"two",3.3333] |
You may also declare and assign several variables at the same time.
1 | Local $x = 1, $y = "", $z[3] = [1,2,3] |
Operators
What Are Operators
In computer programming, operators are constructs defined within programming languages which behave generally like functions, but which differ syntactically or semantically. Common simple examples include arithmetic (e.g. addition with +), comparison (e.g. “greater than” with >), and logical operations (e.g. AND, also written && in some languages). More involved examples include assignment (usually = or :=), field access in a record or object (usually .), and the scope resolution operator (often :: or .). Languages usually define a set of built-in operators, and in some cases allow users to add new meanings to existing operators or even define completely new operators. (Wikipedia)
Operators in AutoIt Programming Language
Assignment
= | Assignment operator. e.g. $var = 5 |
&= | Concatenation assignment. e.g. $var &= ”Hello” equivalent to $var = $var & ”Hello” |
Mathematical Operation
+ | Addition operator. e.g. 10 + 20 |
– | Subtraction operator. e.g. 20 – 10 |
* | Multiplication operator. e.g. 20 * 10 |
/ | Division operator. e.g. 20 / 10 |
^ | Powers operator. e.g. 2 ^ 4 |
+= | Addition assignment. e.g. $var += 1 is equivalent to $var = $var + 1 |
-= | Subtraction assignment. e.g. $var -= 1 is equivalent to $var = $var -1 |
*= | Multiplicative assignment. e.g. $var *= 2 is equivalent to $var = $var * 2 |
/= | Divisive assignment. e.g. $var /= 2 is equivalent to $var = $var / 2 |
Mathematical Comparison
= | Equivalence operator. e.g. If $var = 5 Then … |
== | Equivalence operator. e.g. If $var == 5 Then … |
<> | Not equal operator. e.g. If $var <> 5 Then … |
> | Greater-than operator. e.g. If $var > 5 Then … |
>= | Greater-than-or-Equal-to operator. e.g. If $var >= 5 Then … |
< | Less-than operator. e.g. If $var < 5 Then … |
<= | Less-than-or-Equal-to operator. e.g. If $var <= 5 Then … |
String Comparison
= | Equivalence operator. e.g. If $var = ”Hi” Then … ; case insensitive. |
== | Equivalence operator. e.g. If $var == ”Bye” Then … ; case sensitive. |
<> | Not equivalent operator. e.g. If $var <> ”blah” Then … ; case insensitive. |
Not <> | Not equivalent combinative operator. e.g. If Not $var <> ”blah” Then … ; case sensitive. |
Logical
And | Binary logical And operator. e.g. If $var > 5 And $var < 3 Then … |
Or | Binary logical Or operator. e.g. If $var <> 5 Or $var <> 6 Then … |
Not | Unary inversion operator. e.g. If Not $var > 5 Then … |
Identifiers
What Are Identifiers
In computer languages, identifiers are tokens (also called symbols, but not to be confused with the symbol primitive type) that name the language entities. Some of the kinds of entities an identifier might denote include variables, types, labels, subroutines, and packages. (Wikipedia)
Identifiers in AutoIt Programming Language
Functions in AutoIt are first class objects. Among other things, that means you can assign a function to a variable, pass it around as an argument or return from another function.
Aside from certain specific scope-regarding declaration rules (being that the names of the built-in functions are reserved and of UDFs’ can be overwritten only locally), the names of functions do not have special status in the language.
1 | #include <MsgBoxConstants.au3> |
Optional parameters
Many functions contain optional parameters that can be omitted. If you wish to specify an optional parameter, however, all preceding parameters must be specified!
For example, consider Run ( ”filename”, [”workingdir” [, flag]] ). If you wish to specify the flag, you must specify a workingdir.
When an optional parameter needs to be defined and is preceded by one or more optional parameters, the default value must be given for that parameter. Generally speaking functions should accept the Default keyword when you wish to use the default parameter. See the corresponding optional parameter description for more details.
Many Win___ functions contain an optional parameter ”text”. This parameter is intended to help differentiate between windows that have identical titles.
Success/failure indication
Some functions use the Return value to indicate success/failure, others set the @error flag. Some do both….
If the Return value method is used, the Help file will specify the expected value for success/failure – but the success value is typically non-zero to allow easy to read code…
1 | If SomeUserFunc() Then ;...function worked. |
When the documentation states that the return value = none, AutoIt always returns a value to avoid errors. 1 is usually the value returned, but you should not depend on this return value.
If the @error flag method is used, @error = 0 always indicates success. Other @error values indicate a problem and are defined in the Help file for the function.
1 | Local $sFileRead = FileReadLine("C:someFile.txt") |
If a function uses the @error flag method, the flag should be checked immediately after the function returns as @error will be reset to 0 when entering the next function. No attempt to use or access the function return value should be made if the @error flag has been set as in that case the return value is generally undefined…
Printing Texts In AutoIt Programming Language
Writes data to the STDOUT stream. Some text editors can read this stream as can other programs which may be expecting data on this stream.
ConsoleWrite ( ”data” )
Parameters
data – The data you wish to output. This may either be text or binary.
Return Value
The amount of data written. If writing binary, the number of bytes written, if writing text, the number of characters written.
Remarks
The purpose for this function is to write to the STDOUT stream. Many popular text editors can read this stream. Scripts compiled as Console applications also have a STDOUT stream.
This does not write to a DOS console unless the script is compiled as a console application.
Characters are converted to ANSI before being written.
Binary data is written as-is. It will not be converted to a string. To print the hex representation of binary data, use the String() function to explicitly cast the data to a string.
Related
ConsoleRead, ConsoleWriteError
Example
Example 1
1 2 3 4 5 6 7 8 9 10 11 12 | Local $x = 1 If $x = 1 Then ;Code block EndIf If $x < 2 Then ;Code block ElseIf $x >= 3 Then ;Code block Else ;Code block EndIf |
Conditional Statements
What Are Conditional Statements
In computer science, conditionals (that is, conditional statements, conditional expressions and conditional constructs,) are programming language commands for handling decisions. Specifically, conditionals perform different computations or actions depending on whether a programmer-defined boolean condition evaluates to true or false. In terms of control flow, the decision is always achieved by selectively altering the control flow based on some condition (apart from the case of branch predication).
Conditional Statements in AutoIt Programming Language
Branching is to let the computer compare something and based on the result let it execute a block of code. Autoit has three keywords for identifying branching. They are If, Select and Switch. In addition there are several sub keywords to identify the comparing code and assosiated block of code.
If-ElseIf-Else
When a test returns a true statement then execute code block down to the assosiated ElseIf, Else or EndIf keyword. If Else or Else if was encountered then jump to the EndIf Line.
1 2 3 4 5 6 7 8 9 10 11 12 | Local $x = 1 If $x = 1 Then ;Code block EndIf If $x < 2 Then ;Code block ElseIf $x >= 3 Then ;Code block Else ;Code block EndIf |
There also exists a shorthand method of the If statement:
1 | If true = 1 then MsgBox(1,"Is it true?","Yarp!") |
Additionally, there is the Ternary Operator, which acts as a shorthand conditional statement. The Ternary operator allows a binary choice to be executed without the overhead of an If..Else…EndIf statement:
1 2 | #include <MsgBoxConstants.au3> MsgBox($MB_SYSTEMMODAL, "Result: 1=1", (1 = 1) ? "True" : "False") |
Select
Select is simply a (arguably) more readable version of an if-elseif-else statement.
1 2 3 | For $i = 1 to 10 Step 2; in a For..Next loop, the For variable is automatically declared locally ConsoleWrite($i & @LF) Next |
Switch
The Switch statement contains an interesting difference to most languages. In AutoIt’s case, it is equivalent to the Select statement. Once a block of code is executed the Switch statement exits. Unlike most other languages, a break is not required at the end of each case to avoid trickle-down. This may be considered disadvantageous due to the lack of multi-conditional executions that the Switch statement is generally used for.
1 2 3 4 5 6 7 8 | Switch $var Case 1 ;Code block Case 2 ;Code block Case Else ;Code block EndSwitch |
Loops
What Are Loops
In computer programming, a loop is a sequence of instruction s that is continually repeated until a certain condition is reached. Typically, a certain process is done, such as getting an item of data and changing it, and then some condition is checked such as whether a counter has reached a prescribed number. (https://whatis.techtarget.com/definition/loop)
Loops in AutoIt Programming Language
A loop is used on a code block that we want to execute several times. In the code block we can have variables changing state for each iteration in the loop. AutoIt has four types of loops. For…Next, For Each In, While…Wend and Do … Until
For … Next
1 2 3 | For $i = 1 to 10 Step 2; in a For..Next loop, the For variable is automatically declared locally ConsoleWrite($i & @LF) Next |
For Each In
The For Each In loop is often used for associative arrays however it is often handy when you don’t know the size of your current array. In this case, the syntax and implementation is very similar to javascript which is slightly off the standard. Assume we have an array $arr of undetermined size:
1 2 3 4 5 | Local $str = “” for $stuff in $arr ; for each item in our array $str &= $stuff ; copy the item into our string Next MsgBox(1,””,$str) ; displays the contents of our string |
While … WEnd
1 2 3 4 5 | Local $var While $var < 100 Sleep(100) $var += 1 WEnd |
Do … Until
;A Do .. Until block will run at least once as the conditional check is done at the bottom of the block.
1 2 3 4 5 | Local $var Do Sleep(100) $var += 1 Until $var = 100 |
The code inside this Do … Until block should only run once
1 2 3 4 5 | Local $c Do $c += 1 ConsoleWrite(“Run nr ” & $c & @crlf) Until True |
This sample will run until we forcefully us a ExitLoop command to escape the loop
1 2 3 4 5 6 | Local $c Do $c += 1 ConsoleWrite(“Run nr ” & $c & @crlf) If $c = 2 Then ExitLoop Until False |
This sample should run until $c equals 3
1 2 3 4 5 | Local $c Do $c += 1 ConsoleWrite(“Run nr ” & $c & @crlf) Until $c=3 |
Other Posts
Sources
https://www.autoitscript.com/wiki/Tutorial_Core_Language
https://www.autoitscript.com/autoit3/docs/intro/lang_datatypes.htm
https://www.autoitscript.com/autoit3/docs/functions/ConsoleWrite.htm
https://www.autoitscript.com/autoit3/docs/function_notes.htm
https://en.wikipedia.org/wiki/AutoIt