What is The Difference Between C++ And ICI, Programming Languages
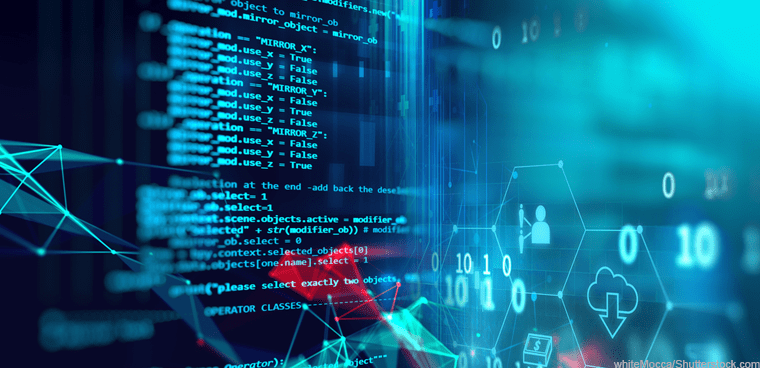
C++ is a Compiled Programming Language, while ICI is an Interpreted Programming Language
What are Compiled Programming Languages
A compiled language is a programming language whose implementations are typically compilers (translators that generate machine code from source code), and not interpreters (step-by-step executors of source code, where no pre-runtime translation takes place). (Wikipedia)
What are Interpreted Programming Languages
An interpreted language is a programming language for which most of its implementations execute instructions directly, without previously compiling a program into machine-language instructions. The interpreter executes the program directly, translating each statement into a sequence of one or more subroutines already compiled into machine code. (Wikipedia)
While C++ is a Compiled Programming Language, and ICI is an Interpreted Programming Language
Let us now look at the difference between the two:
What is C++ Programming Language – A brief synopsis
It consists of a combination of high-level and low-level language features and is hence considered as a middle-level programming language. Bjarne Stroustrup of Bell Labs developed C++ as an extension of the C language. Originally known as ‘C with Classes’, it came to be known as C++ from 1983. It is a multi-paradigm language that supports procedural programming, generic programming, object-oriented programming, and data abstraction.
What is ICI Programming Language – A brief synopsis
Designed by Tim Long in 1992, ICI is a general purpose interpreted computer programming language. It supports dynamic typing, flexible data types and other language constructs similar to C.
Sources
A Complete List of Computer Programming Languages